Spring Framework
The Spring framework is small and light. Because it offers support for multiple frameworks, including Struts, Hibernate, Tapestry, EJB, JSF, and others, it can be viewed as a framework of frameworks. In a more general sense, the framework is a structure that helps us solve different kinds of technological issues.
Folder Structure of Code
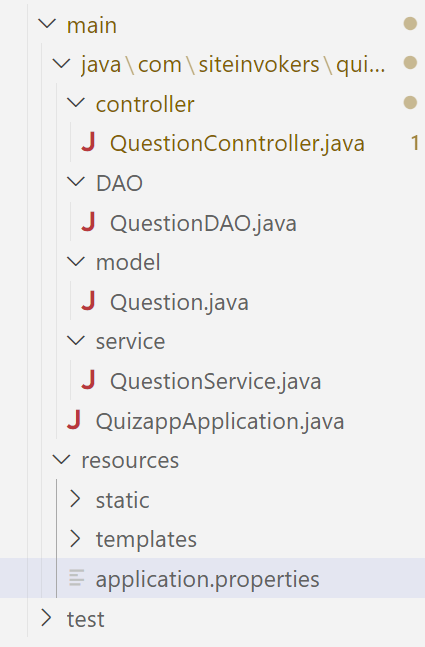
POM file
Code for file pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.1.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.siteinvokers</groupId>
<artifactId>quizapp</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>quizapp</name>
<description>Quizapp using spring boot</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Properties File
Code for file application.properties
spring.datasource.driver-class-name=org.postgresql.Driver
spring.datasource.url=jdbc:postgresql://localhost:5432/questionDB
spring.datasource.username=postgres
spring.datasource.password=123456
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.PostgreSQLDialect
Controller Code
Code for file QuestionConntroller.java
package com.siteinvokers.quizapp.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.siteinvokers.quizapp.model.Question;
import com.siteinvokers.quizapp.service.QuestionService;
@RestController
@RequestMapping("question")
public class QuestionConntroller {
@Autowired
QuestionService questionService;
@GetMapping("allQuestions")
public ResponseEntity<List<Question>> getAllQuestion() {
return questionService.getAllQuestions();
}
@GetMapping("category/{category}")
public ResponseEntity<List<Question>> getQuestionByCategory(@PathVariable String category) {
return questionService.getQuestionByCategory(category);
}
@PostMapping("add")
public ResponseEntity<String> addQuestion(@RequestBody Question question) {
System.out.println("Controller : " + question.toString());
return questionService.addQuestion(question);
}
@DeleteMapping("question/{questionId}")
public ResponseEntity<String> deleteQuestionById(@PathVariable int questionId){
return questionService.deleteQuestionById(questionId) ;
}
@PutMapping("update")
public ResponseEntity<String> updateQuestion(@RequestBody Question question) {
System.out.println("Controller : " + question.toString());
return questionService.addQuestion(question);
}
}
DAO Code
Code for file QuestionDAO.java
package com.siteinvokers.quizapp.DAO;
import java.util.List;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.siteinvokers.quizapp.model.Question;
@Repository
public interface QuestionDAO extends JpaRepository<Question, Integer> {
List<Question> findByCategory(String category);
}
Model Layer Code
Code for file Question.java
In the model layer, we define the database fields which can be seen in our database.
package com.siteinvokers.quizapp.model;
import org.springframework.stereotype.Component;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import lombok.Data;
@Data
@Entity
@Component
public class Question {
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE)
private Integer id;
private String questionTitle;
private String option1;
private String option2;
private String option3;
private String option4;
private String rightAnswer;
private String difficultyLevel;
private String category;
@Override
public String toString() {
return "Question [id=" + id + ", questionTitle=" + questionTitle + ", option1=" + option1 + ", option2="
+ option2 + ", option3=" + option3 + ", option4=" + option4 + ", rightAnswer=" + rightAnswer
+ ", difficultyLevel=" + difficultyLevel + ", category=" + category + "]";
}
}
Service Layer Code
Code for file QuestionService.java
package com.siteinvokers.quizapp.service;
import java.util.ArrayList;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import com.siteinvokers.quizapp.DAO.QuestionDAO;
import com.siteinvokers.quizapp.model.Question;
@Service
public class QuestionService {
@Autowired
QuestionDAO questionDao;
public ResponseEntity<List<Question>> getAllQuestions() {
System.out.println(questionDao.findAll());
try {
return new ResponseEntity<>(questionDao.findAll(), HttpStatus.OK);
} catch (Exception e) {
e.printStackTrace();
}
return new ResponseEntity<>(new ArrayList<>(), HttpStatus.BAD_REQUEST);
}
public ResponseEntity<List<Question>> getQuestionByCategory(String category) {
try{
return new ResponseEntity<>(questionDao.findByCategory(category), HttpStatus.OK);
}catch(Exception e){
e.printStackTrace();
}
return new ResponseEntity<>(new ArrayList<>(), HttpStatus.BAD_REQUEST);
}
public ResponseEntity<String> addQuestion(Question question) {
try{
questionDao.save(question) ;
return new ResponseEntity<String>("Success" , HttpStatus.CREATED);
}catch(Exception e){
e.printStackTrace();
}
return new ResponseEntity<>("Bad Request", HttpStatus.BAD_REQUEST);
}
public ResponseEntity<String> deleteQuestionById(int questionId) {
try{
questionDao.deleteById(questionId) ;
return new ResponseEntity<>("Question id " + questionId + " is deleted from Database" , HttpStatus.OK);
}catch(Exception e){
e.printStackTrace();
}
return new ResponseEntity<>("Not able to delete", HttpStatus.BAD_REQUEST);
}
}
Git Hub Link for Java Spring Boot Project
Related Posts:
Comparison Between Java And Python Programming Languages. Java Spring MVC (Model View Controller) - Model Attribute Java Spring MVC (Model View Controller) Exception Handling In Java Multi-Threading in Java Generics In Java Set Interface in Java List in Java Java Interview Questions (Part 2) Java Annotations Java Viva Questions (Part 1)