In this article we will discuss three different ways to swap two numbers in python programming language.
- Python program to swap two numbers using third variable.
- Python program to swap two numbers without using third variable.
- Python program to swap two numbers in one line.
1.Python program to swap two numbers using third variable.
First, we will swap two numbers using third variable. Suppose we have two boxes so that in first box we kept a and in second box we kept b. So to swap two values we bring third.
Now we will keep a in third box, so now the first box which has a is empty. In first box we will keep b, and then in second box which has b we will again keep a.
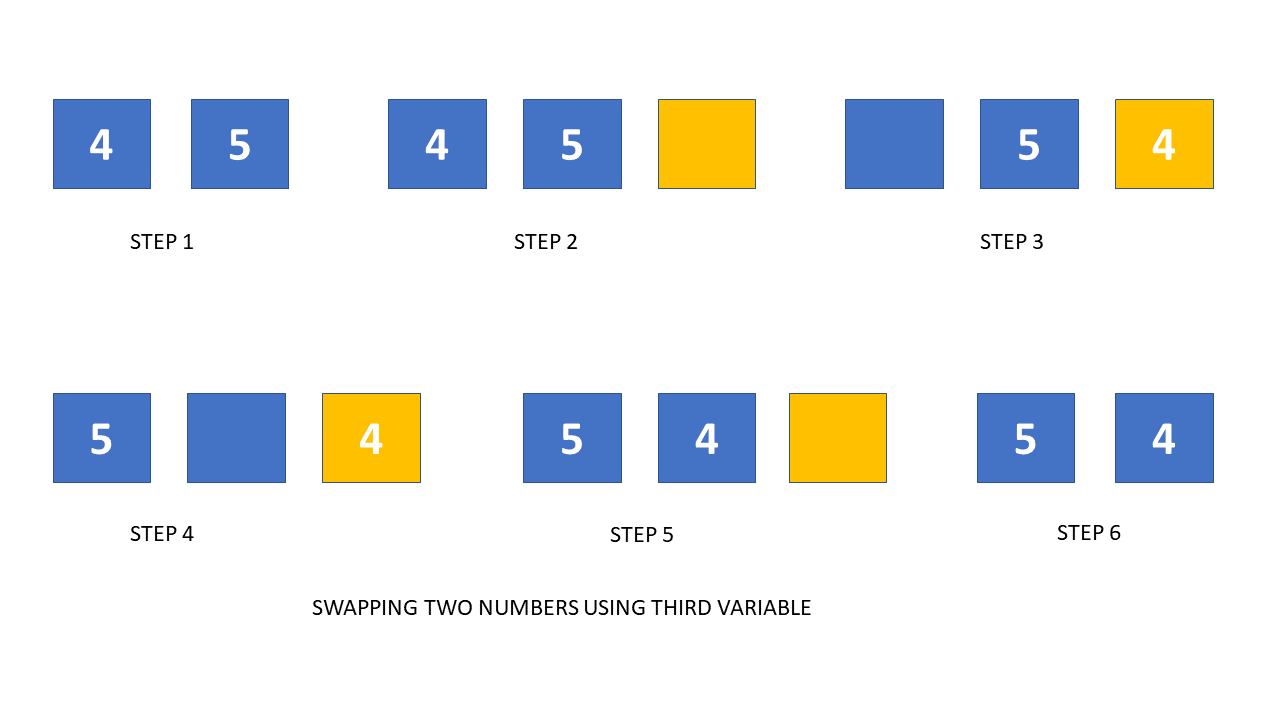
This is how we will swap two numbers in python.
a= int(input("Enter first digit:"))
b= int(input("Enter second digit:"))
temp = a
a=b
b=temp
print("Value of a after swapping:",a)
print("Value of b after swapping:",b)
Output:
Enter first digit:24
Enter second digit:242
Value of a after swapping: 242
Value of b after swapping: 24
2.Python program to swap two numbers without using third variable.
In this method we will swap the numbers without using third variable. First, we will add two numbers and store them in a. Now we will subtract b from a to get swapped value of b, similarly we will subtract new value of b from a to get the swapped value of a.
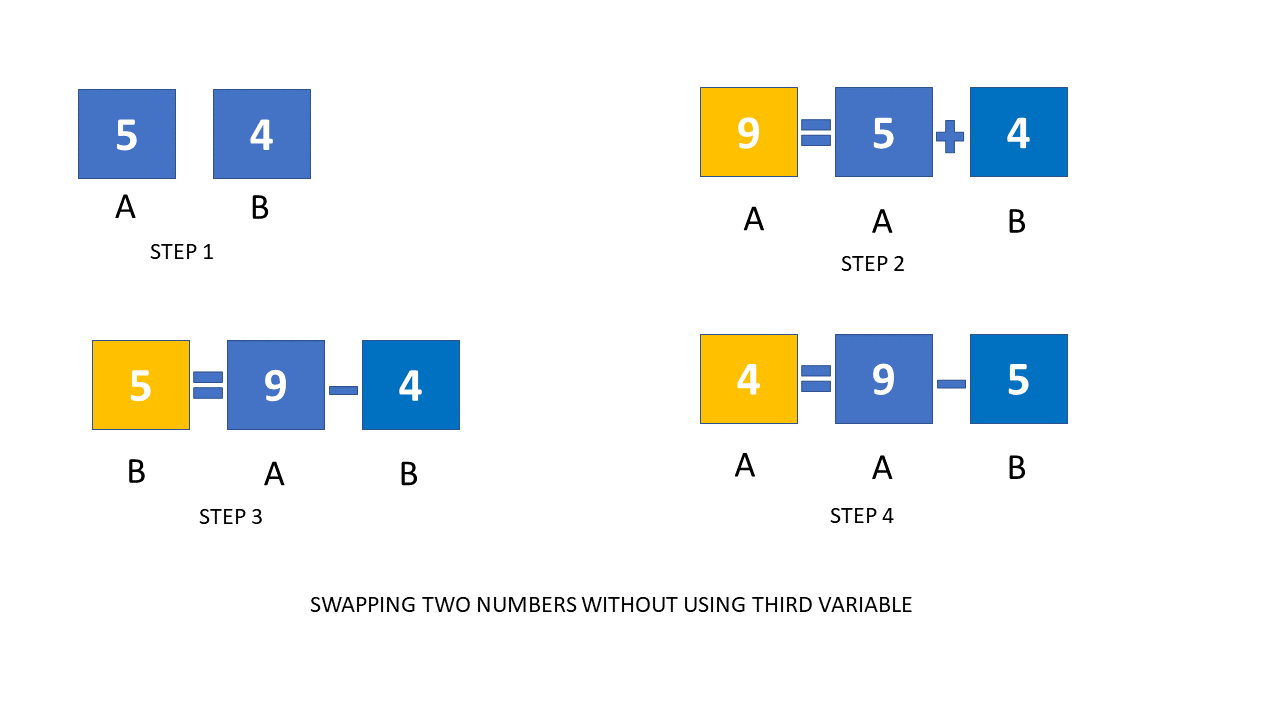
This is how we will swap two numbers in python.
a= int(input("Enter first digit:"))
b= int(input("Enter second digit:"))
a=a+b
b=a-b
a=a-b
print("Value of a after swapping:",a)
print("Value of b after swapping:",b)
Output:
Enter first digit:34
Enter second digit:2424
Value of a after swapping: 2424
Value of b after swapping: 34
3.Python program to swap two numbers in one line.
This is how we will swap two numbers in python.
Output:
a,b= input("Enter two digits:").split()
a,b=b,a
print("Value of a after swapping is {} and Value of b after swapping is {}:".format(a,b))
Output:
Enter two digits:34 12
Value of a after swapping is 12 and Value of b after swapping is 34